朋友的苹果手机爬墙软件不支持订阅链接,每次都要找我要地址,有亿点麻烦。。。
老规矩,请chatgpt大哥帮忙将机场订阅链接解析成可直接导入的服务器地址,并给页面增加一个访问密码,对移动端显示做了优化。修改代码中的访问密码和订阅地址参数后即可使用。
<?php
session_start();
// 设置访问密码
$password = "your_password"; // 将 "your_password" 替换为你的实际密码
// 最大登录尝试次数和锁定时间(单位:秒)
$max_attempts = 3;
$lock_time = 6000; // 60分钟
// 检查是否已通过验证
if (!isset($_SESSION['authenticated'])) {
// 如果用户未通过验证,检查当前的IP
$user_ip = $_SERVER['REMOTE_ADDR'];
// 初始化尝试记录
if (!isset($_SESSION['attempts'])) {
$_SESSION['attempts'] = 0;
$_SESSION['first_attempt_time'] = time();
}
// 检查是否超过最大尝试次数
if ($_SESSION['attempts'] >= $max_attempts) {
// 如果锁定时间尚未到,显示提示信息
if (time() - $_SESSION['first_attempt_time'] < $lock_time) {
$remaining_time = $lock_time - (time() - $_SESSION['first_attempt_time']);
die("您尝试次数过多,请稍后再试。锁定时间: $remaining_time 秒。");
} else {
// 如果锁定时间到,重置尝试次数
$_SESSION['attempts'] = 0;
}
}
// 处理表单提交的密码
if ($_SERVER['REQUEST_METHOD'] === 'POST' && isset($_POST['password'])) {
if ($_POST['password'] === $password) {
// 密码正确,设置会话变量
$_SESSION['authenticated'] = true;
$_SESSION['attempts'] = 0; // 重置尝试次数
} else {
// 密码错误,增加尝试次数
$_SESSION['attempts']++;
$_SESSION['first_attempt_time'] = time(); // 更新首次尝试时间
$error_message = "密码错误,请重试。";
}
}
// 如果未通过验证,显示密码输入表单
if (!isset($_SESSION['authenticated'])) {
?>
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>访问受限</title>
</head>
<body>
<h1>请输入访问密码</h1>
<form method="post">
<label for="password">密码:</label>
<input type="password" name="password" id="password" required>
<button type="submit">提交</button>
</form>
<?php if (isset($error_message)) { echo "<p style='color: red;'>$error_message</p>"; } ?>
</body>
</html>
<?php
exit(); // 停止执行,直到输入正确密码
}
}
// 机场订阅地址
$subscription_url = "替换为机场订阅地址";
// 函数:获取并解析订阅内容
function getV2RayLinks($url) {
// 获取订阅内容
$content = file_get_contents($url);
if ($content === false) {
return "无法获取订阅内容,请检查订阅地址是否正确。";
}
// 解码 Base64 内容
$decoded_content = base64_decode($content);
if ($decoded_content === false) {
return "订阅内容解码失败。";
}
// 去除多余空格并按行排列
$links = array_map('trim', explode("\n", trim($decoded_content)));
return $links;
}
// 处理更新请求
$parsed_links = [];
if ($_SERVER['REQUEST_METHOD'] === 'POST' && isset($_POST['update'])) {
$parsed_links = getV2RayLinks($subscription_url);
}
?>
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>订阅解析</title>
<style>
body {
font-family: Arial, sans-serif;
padding: 10px;
max-width: 600px;
margin: auto;
}
h1, h2 {
text-align: center;
}
.link-container {
display: flex;
justify-content: space-between;
align-items: center;
border: 1px solid #ccc;
padding: 8px;
margin: 5px 0;
border-radius: 5px;
font-size: 14px;
word-break: break-all;
}
.copy-button {
font-size: 12px;
padding: 5px 8px;
background-color: #007bff;
color: #fff;
border: none;
border-radius: 4px;
cursor: pointer;
}
.copy-button:active {
background-color: #0056b3;
}
</style>
</head>
<body>
<h1>订阅解析</h1>
<!-- 更新按钮 -->
<form method="post" style="text-align: center;">
<button type="submit" name="update">更新</button>
</form>
<!-- 显示解析结果 -->
<div>
<h2>解析后的链接:</h2>
<?php if ($parsed_links): ?>
<?php foreach ($parsed_links as $link): ?>
<div class="link-container">
<span class="link-text"><?php echo htmlspecialchars($link); ?></span>
<button class="copy-button" onclick="copyToClipboard('<?php echo htmlspecialchars($link); ?>')">复制</button>
</div>
<?php endforeach; ?>
<?php else: ?>
<p style="text-align: center;">点击上方的“更新”按钮获取最新链接</p>
<?php endif; ?>
</div>
<script>
function copyToClipboard(link) {
// 创建临时元素用于复制
const tempInput = document.createElement("textarea");
tempInput.value = link;
document.body.appendChild(tempInput);
tempInput.select();
tempInput.setSelectionRange(0, 99999); // 兼容移动端
document.execCommand("copy");
document.body.removeChild(tempInput);
alert("已复制链接到剪贴板");
}
</script>
</body>
</html>
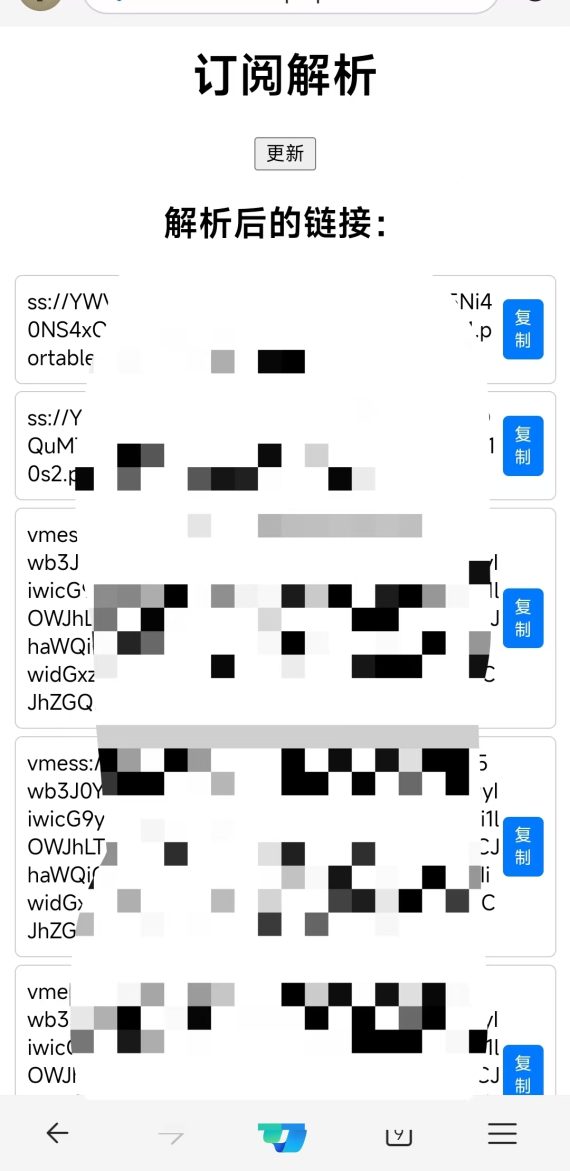